In this post, we delve into the differences between Map and HashMap in Java to gain a deeper understanding of their characteristics.
HashMap is a class within the Java Collection Framework that does not offer synchronization and accommodates both null values and keys. In contrast, Map is an interface in Java utilized for mapping key-value pairs. While both provide key-value mapping and a means to iterate over keys, their key distinctions revolve around timing guarantees and key order.
How Does Map Differ from HashMap in Java?
- Insertion Order: HashMap does not preserve the insertion order of elements, making it faster compared to Map.
- Duplicate Values: Unlike Map, HashMap can store duplicate values.
- Interface vs. Class: HashMap is the implementation of the Map interface; thus, HashMap is all about implementing the Map Interface.
- Null Key: A map does not allow the storage of a single null key, whereas HashMap can store multiple null values along with a single null key.
- Synchronization: Map is an interface, while HashMap is a non-synchronized class within the Java Collections framework.
HashMap Methods:
computeIfAbsent(K key, Function<? super K,? extends V> mappingFunction)
: Calculates and enters the value for the provided key using a given mapping function if it is not already associated with a value.clear()
: Removes all mappings from the HashMap.containsValue(Object value)
: Checks if the HashMap maps one or more keys to the specified value.compute(K key, BiFunction<? super K, ? super V,? extends V> remappingFunction)
: Computes a mapping for the specified key based on its current mapped value or null if no mapping exists.clone()
: Creates a shallow clone of the HashMap object, retaining keys and values.computeIfPresent(K key, BiFunction<? super K, ? super V,? extends V> remappingFunction)
: Computes a new mapping if the specified key exists and has a current mapped value.containsKey(Object key)
: Determines if the HashMap has a mapping for the given key.entrySet()
: Constructs a set of elements contained in a HashMap.get(Object key)
: Retrieves the value mapped to the provided key or null.values()
: Constructs a collection of the HashMap’s values.
Map Interface Methods:
get(Object)
: Retrieves the value mapped to a specified key.hashCode()
: Returns the hash code of a string containing the key and value for the given map.equals(Object)
: Compares two maps to determine their similarity.put(Object, Object)
: Links a value to a specified key.putAll(Map)
: Copies all mappings from one map to another.isEmpty()
: Checks if the map is empty.size()
: Returns the number of key-value pairs in the map.values()
: Constructs a collection from the map’s values.keySet()
: Provides a Set view of the keys in the map, allowing changes to be automatically reflected.clear()
: Clears all elements or mappings from the map.containsKey(Object)
: Determines if a given key is mapped in the Map.containsValue(Object)
: Checks if a given value is mapped by one or more keys.entrySet()
: Produces a set from the map’s elements or creates a new set to store map entries.
Using HashMap Class Directly
// Declare a HashMap
HashMap<String, Integer> hashMap = new HashMap<>();
// Adding key-value pairs to the HashMap
hashMap.put("apple", 5);
hashMap.put("banana", 3);
hashMap.put("cherry", 7);
// Retrieving values from the HashMap
int count = hashMap.get("banana"); // Returns 3
// HashMap specific method - Getting all values
Collection<Integer> values = hashMap.values();
// Checking if a key exists in the HashMap
boolean containsKey = hashMap.containsKey("grape"); // Returns false
// Size of the HashMap
int size = hashMap.size(); // Returns 3
// Iterating over key-value pairs
for (Map.Entry<String, Integer> entry : hashMap.entrySet()) {
System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue());
}
// Clearing the HashMap
hashMap.clear();
In Summary
Map and HashMap share similarities, but their distinction lies in the interface. HashMap operates as an interface in the case of HashMap, whereas Map operates as Map. HashMap represents a dynamic form of Map, while Map is a static type of Map. Consequently, you can change the dynamic type of Map at runtime as long as it remains a subtype of Map without violating the contract with existing code. This flexibility is a significant advantage of using Map. Conversely, if declared as HashMap, a contract modification would be necessary when altering the underlying implementation.
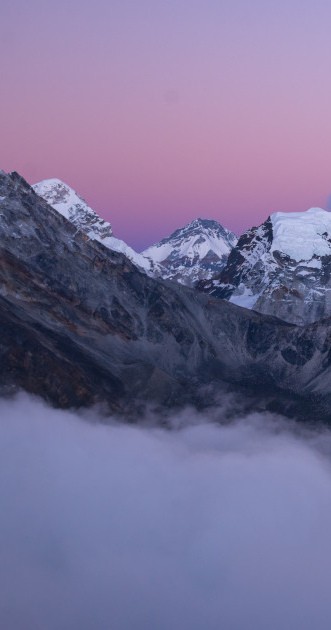
ERPixel is Odoo development company
Our Services:
Ready to supercharge your digital transformation? Discover top-notch Odoo development services from ERPixel.
Take the first step:
Programming against interfaces rather than implementations provides flexibility and the ability to change the map’s behavior on the fly. There are many more differences between Map and HashMap in Java, and you can reach out to our Ksolves experts for further insights. We specialize in Java programming and are experts in Java/J2EE development using jQuery.